Apply Law's texture 2d filter on an image.
Law's texture filter generates texture features to quantify the perceived 2D textures of an image.
Law's texture 2D filter involves the following steps:
- pre-process phasis: subtract mean neighborhood intensity from each pixel of input image to obtain a zero-mean image and thus to remove effects of illumination. By default, the algorithm subtracts the mean of a square neighborhood of size 15x15, but, depending on the provided pre-process parameters, the user has also the possibility to change the size of the square neighborhood, or to subtract a gaussian smoothed neighborhood instead).
filter the neighborhood by applying convolutions with the 15 5x5 Law's masks; Law's masks are built from 4 1D kernels:
First letters of the names of these 1D kernels respectively stand for:
- Level detector,
- Edges detector,
- Spots detector,
- Ripples detector.
The 15 5x5 Law's masks are obtained by multiplying the 4 1D kernels with their 5 transpose kernels (the 16th, L5L5, is not computed, because it's only a smoothing mask and it does not contain any real texture information). For instance, one of the 15 masks, E5L5, is computed as follows:

The 15 5x5 Law's masks are named as follows: 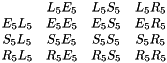
All these masks are zero-sum masks.
- Compute the 15 energy images by either, depending on specified post-process parameters:
- for each filtered pixel, averaging the absolute values across a square neigborhood of a given size around pixel (this is the default mode, with a default kernel half-size of 7),
- for each filtered pixel, averaging the values of filtered images across a square neigborhood of a given size around pixel,
- for each filtered pixel, smoothing the absolute values of neighborhood with a gaussian kernel,
- for each filtered pixel, smoothing the values of neighborhood with a gaussian kernel,
- for each filtered pixel, computed the variance of a square neighborhood around pixel.
Compute the 9 final energy maps, in order:
Each pair is replaced with the average of the 2 images.
Resulting energy maps are concatenated in the output sequence image in the order specified above. Most of the time, only a subset of the 9 energy maps are interesting. The user has the possibility to specify this subset by appropriately initializing a ipsdk::imaproc::attr::LawTextureKernel2dTypes data-item and passing it as argument of lawTexture2dImg function. Then only the maps of this subset will be calculated by the algorithm and returned to the user.
Here is an example of a Law's 2D Texture Filter computation applied on a 8 bits grey level image with the default parameters:
Example of Python code :
Example imports
import PyIPSDK
import PyIPSDK.IPSDKIPLStats as stats
Code Example
inImg = PyIPSDK.loadTiffImageFile(inputImgPath)
outImg = stats.lawTexture2dImg(inImg)
outImgE5E5 = PyIPSDK.extractPlan(0, 0, 3, outImg)
preProcParams = PyIPSDK.createGaussianLawTexPreProcParams(0.7, PyIPSDK.createGaussianCoverage(0.991, 2))
postProcParams = PyIPSDK.createVarianceLawTexPostProcParams(8)
kernelTypes = PyIPSDK.createLawTextureKernel2dTypes()
kernelTypes.flagL5E5_E5L5 = False
kernelTypes.flagL5S5_S5L5 = False
kernelTypes.flagL5R5_R5L5 = False
kernelTypes.flagE5S5_S5E5 = False
kernelTypes.flagE5R5_R5E5 = False
kernelTypes.flagS5S5 = False
kernelTypes.flagS5R5_R5S5 = False
outImg = stats.lawTexture2dImg(inImg, kernelTypes, preProcParams, postProcParams);
outImgE5E5 = PyIPSDK.extractPlan(0, 0, 0, outImg)
outImgR5R5 = PyIPSDK.extractPlan(0, 0, 0, outImg)
Example of C++ code :
Example informations
Header file
#include <IPSDKIPL/IPSDKIPLStats/Processor/LawTexture2dImg/LawTexture2dImg.h>
Code Example
{
ImagePtr pOutImg = stats::lawTexture2dImg(pInImg);
ImagePtr pOutImgE5E5;
}
{
pKernelTypes->setValue<LawTextureKernel2dTypes::FlagL5E5_E5L5>(false);
pKernelTypes->setValue<LawTextureKernel2dTypes::FlagL5S5_S5L5>(false);
pKernelTypes->setValue<LawTextureKernel2dTypes::FlagL5R5_R5L5>(false);
pKernelTypes->setValue<LawTextureKernel2dTypes::FlagE5S5_S5E5>(false);
pKernelTypes->setValue<LawTextureKernel2dTypes::FlagE5R5_R5E5>(false);
pKernelTypes->setValue<LawTextureKernel2dTypes::FlagS5S5>(false);
pKernelTypes->setValue<LawTextureKernel2dTypes::FlagS5R5_R5S5>(false);
ImagePtr pOutImg = stats::lawTexture2dImg(pInImg, pKernelTypes, pPreProcParams, pPostProcParams);
ImagePtr pOutImgE5E5;
ImagePtr pOutImgR5R5;
}